mirror of
https://github.com/starr-dusT/citra.git
synced 2024-10-02 10:26:17 -07:00
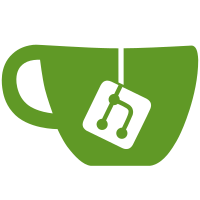
* rasterizer_cache: Switch to template * Eliminates all opengl references in the rasterizer cache headers thus completing the backend abstraction * rasterizer_cache: Switch to page table * Surface storage isn't particularly interval sensitive so we can use a page table to make it faster * rasterizer_cache: Move sampler management out of rasterizer cache * rasterizer_cache: Remove shared_ptr usage * Switches to yuzu's slot vector for improved memory locality. * rasterizer_cache: Rework reinterpretation lookup * citra_qt: Per game texture filter * rasterizer_cache: Log additional settings * gl_texture_runtime: Resolve shadow map comment * rasterizer_cache: Don't use float for viewport * gl_texture_runtime: Fix custom allocation recycling * rasterizer_cache: Minor cleanups * Cleanup texture cubes when all the faces have been unregistered from the cache * custom_tex_manager: Allow multiple hash mappings per texture * code: Move slot vector to common * rasterizer_cache: Prevent texture cube crashes * rasterizer_cache: Improve mipmap validation * CanSubRect now works properly when validating multi-level surfaces, for example Dark Moon validates a 4 level surface from a 3 level one and it works * gl_blit_handler: Unbind sampler on reinterpretation
103 lines
2.2 KiB
C++
103 lines
2.2 KiB
C++
// Copyright 2023 Citra Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <array>
|
|
#include <atomic>
|
|
#include <mutex>
|
|
#include <span>
|
|
#include <string>
|
|
#include <vector>
|
|
#include "video_core/custom_textures/custom_format.h"
|
|
|
|
namespace Frontend {
|
|
class ImageInterface;
|
|
}
|
|
|
|
namespace VideoCore {
|
|
|
|
enum class MapType : u32 {
|
|
Color = 0,
|
|
Normal = 1,
|
|
MapCount = 2,
|
|
};
|
|
constexpr std::size_t MAX_MAPS = static_cast<std::size_t>(MapType::MapCount);
|
|
|
|
enum class DecodeState : u32 {
|
|
None = 0,
|
|
Pending = 1,
|
|
Decoded = 2,
|
|
Failed = 3,
|
|
};
|
|
|
|
class CustomTexture {
|
|
public:
|
|
explicit CustomTexture(Frontend::ImageInterface& image_interface);
|
|
~CustomTexture();
|
|
|
|
void LoadFromDisk(bool flip_png);
|
|
|
|
[[nodiscard]] bool IsParsed() const noexcept {
|
|
return file_format != CustomFileFormat::None && !hashes.empty();
|
|
}
|
|
|
|
[[nodiscard]] bool IsLoaded() const noexcept {
|
|
return !data.empty();
|
|
}
|
|
|
|
private:
|
|
void LoadPNG(std::span<const u8> input, bool flip_png);
|
|
|
|
void LoadDDS(std::span<const u8> input);
|
|
|
|
public:
|
|
Frontend::ImageInterface& image_interface;
|
|
std::string path;
|
|
u32 width;
|
|
u32 height;
|
|
std::vector<u64> hashes;
|
|
std::mutex decode_mutex;
|
|
CustomPixelFormat format;
|
|
CustomFileFormat file_format;
|
|
std::vector<u8> data;
|
|
MapType type;
|
|
};
|
|
|
|
struct Material {
|
|
u32 width;
|
|
u32 height;
|
|
u64 size;
|
|
u64 hash;
|
|
CustomPixelFormat format;
|
|
std::array<CustomTexture*, MAX_MAPS> textures;
|
|
std::atomic<DecodeState> state{};
|
|
|
|
void LoadFromDisk(bool flip_png) noexcept;
|
|
|
|
void AddMapTexture(CustomTexture* texture) noexcept;
|
|
|
|
[[nodiscard]] CustomTexture* Map(MapType type) const noexcept {
|
|
return textures.at(static_cast<std::size_t>(type));
|
|
}
|
|
|
|
[[nodiscard]] bool IsPending() const noexcept {
|
|
return state == DecodeState::Pending;
|
|
}
|
|
|
|
[[nodiscard]] bool IsFailed() const noexcept {
|
|
return state == DecodeState::Failed;
|
|
}
|
|
|
|
[[nodiscard]] bool IsDecoded() const noexcept {
|
|
return state == DecodeState::Decoded;
|
|
}
|
|
|
|
[[nodiscard]] bool IsUnloaded() const noexcept {
|
|
return state == DecodeState::None;
|
|
}
|
|
};
|
|
|
|
} // namespace VideoCore
|