mirror of
https://github.com/starr-dusT/citra.git
synced 2024-10-02 10:26:17 -07:00
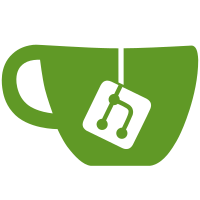
This widget provides a simple list of recorded requests as well as a simple filter and the 'Clear' button. Requests with status 'HLE Unimplemented' or 'Error' will be marked out with the red color. This widget handles retrival of service name. For reasons refer to a previous commit message. Added the widget to the Debugging menu, pretty much the same as other debugging widgets.
53 lines
1.5 KiB
C++
53 lines
1.5 KiB
C++
// Copyright 2019 Citra Emulator Project
|
|
// Licensed under GPLv2 or any later version
|
|
// Refer to the license.txt file included.
|
|
|
|
#pragma once
|
|
|
|
#include <map>
|
|
#include <memory>
|
|
#include <unordered_map>
|
|
#include <QDockWidget>
|
|
#include "core/hle/kernel/ipc_debugger/recorder.h"
|
|
|
|
class QTreeWidgetItem;
|
|
|
|
namespace Ui {
|
|
class IPCRecorder;
|
|
}
|
|
|
|
class IPCRecorderWidget : public QDockWidget {
|
|
Q_OBJECT
|
|
|
|
public:
|
|
explicit IPCRecorderWidget(QWidget* parent = nullptr);
|
|
~IPCRecorderWidget();
|
|
|
|
void OnEmulationStarting();
|
|
|
|
signals:
|
|
void EntryUpdated(IPCDebugger::RequestRecord record);
|
|
|
|
private:
|
|
QString GetStatusStr(const IPCDebugger::RequestRecord& record) const;
|
|
void OnEntryUpdated(IPCDebugger::RequestRecord record);
|
|
void SetEnabled(bool enabled);
|
|
void Clear();
|
|
void ApplyFilter(int index);
|
|
void ApplyFilterToAll();
|
|
QString GetServiceName(const IPCDebugger::RequestRecord& record) const;
|
|
QString GetFunctionName(const IPCDebugger::RequestRecord& record) const;
|
|
void OpenRecordDialog(QTreeWidgetItem* item, int column);
|
|
|
|
std::unique_ptr<Ui::IPCRecorder> ui;
|
|
IPCDebugger::CallbackHandle handle;
|
|
|
|
// The offset between record id and row id, assuming record ids are assigned
|
|
// continuously and only the 'Clear' action can be performed, this is enough.
|
|
// The initial value is 1, which means record 1 = row 0.
|
|
int id_offset = 1;
|
|
std::vector<IPCDebugger::RequestRecord> records;
|
|
};
|
|
|
|
Q_DECLARE_METATYPE(IPCDebugger::RequestRecord);
|