mirror of
https://github.com/ryujinx-mirror/ryujinx.git
synced 2024-10-02 16:50:20 -07:00
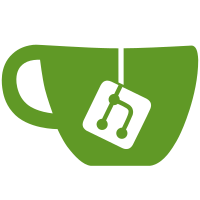
* dotnet format style --severity info Some changes were manually reverted. * dotnet format analyzers --serverity info Some changes have been minimally adapted. * Restore a few unused methods and variables * Address dotnet format CA1816 warnings * Address or silence dotnet format CA2208 warnings * Address or silence dotnet format CA1806 and a few CA1854 warnings * Address dotnet format CA1822 warnings * Make dotnet format succeed in style mode * Address dotnet format CA2208 warnings properly * Address most dotnet format whitespace warnings * Apply dotnet format whitespace formatting A few of them have been manually reverted and the corresponding warning was silenced * Format if-blocks correctly * Another rebase, another dotnet format run * Run dotnet format whitespace after rebase * Run dotnet format after rebase and remove unused usings - analyzers - style - whitespace * Add comments to disabled warnings * Simplify properties and array initialization, Use const when possible, Remove trailing commas * Revert "Simplify properties and array initialization, Use const when possible, Remove trailing commas" This reverts commit 9462e4136c0a2100dc28b20cf9542e06790aa67e. * dotnet format whitespace after rebase * First dotnet format pass * Fix build issues * Apply suggestions from code review Co-authored-by: Ac_K <Acoustik666@gmail.com> * Second dotnet format pass * Update src/Ryujinx/Modules/Updater/Updater.cs Co-authored-by: Ac_K <Acoustik666@gmail.com> * Add trailing commas and improve formatting * Fix formatting and naming issues * Rename nvStutterWorkaround to nvidiaStutterWorkaround * Use using declarations and extend resource lifetimes * Fix GTK issues * Add formatting for generated files * Add trailing commas --------- Co-authored-by: Ac_K <Acoustik666@gmail.com>
48 lines
1.3 KiB
C#
48 lines
1.3 KiB
C#
using OpenTK.Graphics.OpenGL;
|
|
using Ryujinx.Graphics.OpenGL;
|
|
using SPB.Graphics;
|
|
using SPB.Graphics.OpenGL;
|
|
using SPB.Platform;
|
|
using SPB.Windowing;
|
|
|
|
namespace Ryujinx.Ui
|
|
{
|
|
class SPBOpenGLContext : IOpenGLContext
|
|
{
|
|
private readonly OpenGLContextBase _context;
|
|
private readonly NativeWindowBase _window;
|
|
|
|
private SPBOpenGLContext(OpenGLContextBase context, NativeWindowBase window)
|
|
{
|
|
_context = context;
|
|
_window = window;
|
|
}
|
|
|
|
public void Dispose()
|
|
{
|
|
_context.Dispose();
|
|
_window.Dispose();
|
|
}
|
|
|
|
public void MakeCurrent()
|
|
{
|
|
_context.MakeCurrent(_window);
|
|
}
|
|
|
|
public static SPBOpenGLContext CreateBackgroundContext(OpenGLContextBase sharedContext)
|
|
{
|
|
OpenGLContextBase context = PlatformHelper.CreateOpenGLContext(FramebufferFormat.Default, 3, 3, OpenGLContextFlags.Compat, true, sharedContext);
|
|
NativeWindowBase window = PlatformHelper.CreateOpenGLWindow(FramebufferFormat.Default, 0, 0, 100, 100);
|
|
|
|
context.Initialize(window);
|
|
context.MakeCurrent(window);
|
|
|
|
GL.LoadBindings(new OpenToolkitBindingsContext(context));
|
|
|
|
context.MakeCurrent(null);
|
|
|
|
return new SPBOpenGLContext(context, window);
|
|
}
|
|
}
|
|
}
|