mirror of
https://github.com/ryujinx-mirror/ryujinx.git
synced 2024-10-02 16:50:20 -07:00
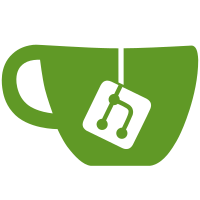
* Implement a new JIT for Arm devices * Auto-format * Make a lot of Assembler members read-only * More read-only * Fix more warnings * ObjectDisposedException.ThrowIf * New JIT cache for platforms that enforce W^X, currently unused * Remove unused using * Fix assert * Pass memory manager type around * Safe memory manager mode support + other improvements * Actual safe memory manager mode masking support * PR feedback
44 lines
1.3 KiB
C#
44 lines
1.3 KiB
C#
using System.Diagnostics;
|
|
|
|
namespace Ryujinx.Cpu.LightningJit.Arm32.Target.Arm64
|
|
{
|
|
static class InstEmitNeonCrypto
|
|
{
|
|
public static void Aesd(CodeGenContext context, uint rd, uint rm, uint size)
|
|
{
|
|
// TODO: Feature check, emulation if not supported.
|
|
|
|
Debug.Assert(size == 0);
|
|
|
|
InstEmitNeonCommon.EmitVectorUnary(context, rd, rm, context.Arm64Assembler.Aesd);
|
|
}
|
|
|
|
public static void Aese(CodeGenContext context, uint rd, uint rm, uint size)
|
|
{
|
|
// TODO: Feature check, emulation if not supported.
|
|
|
|
Debug.Assert(size == 0);
|
|
|
|
InstEmitNeonCommon.EmitVectorUnary(context, rd, rm, context.Arm64Assembler.Aese);
|
|
}
|
|
|
|
public static void Aesimc(CodeGenContext context, uint rd, uint rm, uint size)
|
|
{
|
|
// TODO: Feature check, emulation if not supported.
|
|
|
|
Debug.Assert(size == 0);
|
|
|
|
InstEmitNeonCommon.EmitVectorUnary(context, rd, rm, context.Arm64Assembler.Aesimc);
|
|
}
|
|
|
|
public static void Aesmc(CodeGenContext context, uint rd, uint rm, uint size)
|
|
{
|
|
// TODO: Feature check, emulation if not supported.
|
|
|
|
Debug.Assert(size == 0);
|
|
|
|
InstEmitNeonCommon.EmitVectorUnary(context, rd, rm, context.Arm64Assembler.Aesmc);
|
|
}
|
|
}
|
|
}
|